ElementCollection (class)
Overview
Summary
The ElementCollection class is designed to group and manipulate elements on the Flash stage.
It is a subclass of the Collection class, background information about which can be found in the Introduction to Collections article.
Contents
- Overview
-
API
Usage
The ElementCollection class expects an array of stage elements as its only parameter, so is nearly always used in conjunction with the Element Selector function (although you can of course pass in elements manually). This allows you to abstract fairly complex selections into an easy-to-manage expressions.
The following example creates an ElementCollection using standard dom properties:
var collection = new ElementCollection($selection); collection.list();
[object ElementCollection length=5]: Array (depth:1, objects:0, values:12, time:0.0 seconds) -------------------------------------------------------------------------------------------- array => Array 0: "Item_12" 1: "Item_13" 2: "Item_14" 3: "Item_15" 4: "Item_16"
The following example uses the Element Selector function to grab the current stage selection:
var collection = $(':selection'); collection.list();
[object ElementCollection length=5]: Array (depth:1, objects:0, values:12, time:0.0 seconds) -------------------------------------------------------------------------------------------- array => Array 0: "Item_12" 1: "Item_13" 2: "Item_14" 3: "Item_15" 4: "Item_16"
Note that a collection does not select the items on the stage - it merely holds a reference to them. To physically select items on the stage, see the select() method.
ElementCollection extends Collection
One last note - remember that ElementCollection extends Collection, so you still get to use all the methods on the Collection class, including each(), which is a very powerful method for applying custom manipulation to collection elements:
See the example in Working with Elements for more infomation.
Running the example code
If you want some elements to to quickly test the example code on this page, run the following code which will create some transparent red squares on the Flash stage:
function makeSquares(num, cols) { // variables num = num || 9; cols = cols || 3; var dom = window.dom; var lib = dom.library; var context = Context.create(); // make square if( ! lib.itemExists('square')) { lib.addNewItem('movie clip', 'square'); lib.editItem('square'); dom.addNewRectangle({left:-25, top:-25, right:25, bottom:25}, 0); dom.selectAll(); dom.setFillColor('#FF000066'); dom.setStroke('#000000', 0.5, 'solid') context.goto(); } // add items to scene var collection = $('*'); for(var i = 0; i
API
ElementCollection(elements, dom)
ElementCollection class enacpsulates and modifies Arrays of stage Elements
The following example grabs all items on the stage:
var collection = $('*'); collection.list();[object ElementCollection length=10]: Array (depth:1, objects:0, values:10, time:0.0 seconds) --------------------------------------------------------------------------------------------- array => Array 0: "Item_01" 1: "Item_02" 2: "Item_03" 3: "Item_04" 4: "Item_05" 5: "Item_06" 6: "Item_07" 7: "Item_08" 8: "Item_09" 9: "Item_10"Properties
items
Returns an ItemCollection of the collection's elements
The items property gives you direct access to the Library Items that are used in the current collection, by way of an ItemCollection.
The following example first lists the current elements, then lists and reveals the corresponding Library Items in the Library panel:
$($selection) // create the collection .list() // list the elements .items // return an ItemCollection from the ElementCollection .list() // list the items .reveal(); // and finally, reveal them in the Library[object ElementCollection length=9]: Array (depth:1, objects:0, values:9, time:0.0 seconds) ------------------------------------------------------------------------------------------- array => Array 0: "name: square1" 1: "name: square2" 2: "name: star1" 3: "name: star2" 4: "name: triangle1" 5: "name: square3" 6: "name: star3" 7: "name: circle4" 8: "name: square4" [object ItemCollection length=4]: Array (depth:1, objects:0, values:4, time:0.0 seconds) ---------------------------------------------------------------------------------------- array => Array 0: "2 - elements/shapes/square" 1: "2 - elements/shapes/star" 2: "2 - elements/shapes/triangle" 3: "2 - elements/shapes/circle
Inherited members
ElementCollection is a subclass of the Collection class:
Standard methods
select(element)
Select one or all of the elements within the collection
The following example selects all (unlocked) items on the stage:
$('*').select();
The following example selects the item named "Item_03" in the collection:
$('*').select('Item_05');
The following example selects each of the items in turn, and alerts its progress:
function select(element, index) { this.select(index); alert('Selected ' + element.name) } $('*').each(select);
Manipulation methods
The manipulation methods add or remove items from the collection.
duplicate(add)
Duplicates and updates the current collection
The following example creates some squares, then chains up duplicate and move commands to create an array of elements:
makeSquares(5, 5); $('*') .space('horizontal', 10) .move(10, 10) .duplicate() .move(0, 60, true) .duplicate() .move(0, 60, true)
Note that new items are automatically renamed from their existing names and numbers. In the above example, the newly-duplicated items were labeled based on from the highest-numbered item in the previous element, Item_05, creating Item_06 through to Item_15.
deleteElements()
Removes all elements from the collection and the stage
The following example deletes the selected elements:
$(':selected').deleteElements();
Translation methods
The translation methods modify the geometry of the elements, either as a whole, or indivdually.
- move(x, y, relative)
- rotate(angle, whichCorner)
- scale(xScale, yScale, whichCorner)
- centerTransformPoint(state)
- resetTransform()
move(x, y, relative)
Move the collection on stage by, or to, x and y values
The following example moves all elements of the collection, as a whole unit, to the top-left hand corner of the stage, with the bounding box of the collection aligned to the [0,0] point:
$('*').move(0, 0);
rotate(angle, whichCorner)
Rotates the collection by a specified number of degrees
Note that the rotate method currently uses Flash's rotateSelection() method, so will select the elements before rotating them. This may change in a later release.
The following example rotates the current selection by 45 degrees:
$($selection).rotate(45);
scale(xScale, yScale, whichCorner)
Scales the collection by a specified amount
Note that the scale method currently uses Flash's scaleSelection() method, so will select the elements before scaling them. This may change in a later release.
The following example scales the current selection by 50%:
$($selection).scale(0.5, 0.5);
centerTransformPoint(state)
Centers the transform points of the elements
The following example centers the transform point of the current selection:
$($selection).centerTransformPoint();
resetTransform()
Resets the transform of the elements
The following example rotates the stage elements, then after the alert, clears the transformation:
$('*') .attr('rotation', 45) .call(alert, 'Click OK to reset transform') .resetTransform();
Attribute methods
The attribute methods modify some attribute, or group of attributes of each of the elements.
- attr(prop, value)
- rename(baseName, padding, startIndex, separator)
- orderBy(prop, reverseOrder)
- align(props, element)
- distribute(props, toStage)
- space(direction, type)
- match(prop, element)
- toGrid(precision, rounding)
- randomize(prop, modifier)
attr(name, value)
Sets a single property on each element in the collection
The attr() method is identical to the Collection attr() method with the subtle difference that it supports the following compound pseudo-properties:
- position [x, y] x and y in stage coordinatesy
- scale [scaleX, scaleY] Pass in a single number to set scale for both axis
- size [width, height] Sets the width and height in stage (not element) space, so will skew the object if rotated
You can supply either a single Number to copy the value to both components (ie, scaleX and scaleY), and Array of 2 values, or an object with x and y properties.
Note that attr() also handles alpha, tint, and brightness, but you need to select() the elements first. See the nth() example on the Selectors page for more info.
The following example sets the rotation property of all objects:
$('*').attr('rotation', 30);
The following example sets the x and y scale of all the objects:
$('*').attr('scale', [1, 0.5]);
The following 2 examples update 2 properties at the same time, as well as using a callback, to set a random position on the collection's elements:
function rand(){ return Math.random() * 200 - 100; } // function is called twice $('*').attr({x:rand, y:rand});
function rand(){ return [Math.random() * 200, Math.random() * 200]; } // function passes back a 2-element array $('*').attr('position', rand);
This example sets the scale of an object using a compound property name (scale) but passes back only a single value, which is set for both components (scaleX & scaleY):
function rand(){ return Math.random() + 0.5; } $('*').attr('scale', rand);
rename(baseName, startIndex, padding, separator)
Numerically renames elements in order
The following example performs a default rename, renaming all items on the stage from the starting index "1" padding as necessary:
$('*') .rename() .list();
[object ElementCollection length=10]: Array (depth:1, objects:0, values:10, time:0.0 seconds) --------------------------------------------------------------------------------------------- array => Array 0: "Item_01" 1: "Item_02" 2: "Item_03" 3: "Item_04" 4: "Item_05" 5: "Item_06" 6: "Item_07" 7: "Item_08" 8: "Item_09" 9: "Item_10"
The following examples demonstrate various renaming techniques:
var items = $(':selection');
items.rename(); // rename using defaults, "clip_1", "clip_2", "clip_3" ... items.rename('clip_##'); // rename, starting from 1, padding to 2 characters, "clip_01", "clip_02", "clip_03" ... items.rename('clip_025'); // rename, starting from 25, padding to 3 characters, "clip_025", "clip_026", "clip_027" ... items.rename('clip', 4, 25); // rename, starting from 25, padding to 4 characters, "clip_0025", "clip_0026", "clip_0027" ...
The following example uses a callback to rename any symbols on stage with their class name:
$$(':symbol').rename( function(element){ return element.libraryItem.linkageClassName.split('.').pop(); } );
orderBy(prop, reverseOrder)
Reorder the elements on the stage (front-to-back) by an arbitrary property
The following example positions, scales, then orders elements in reverse order by size, a pseudo-property derived from width x height (note that orderBy() also reorders the elements within the internal Array, which will affect subsequent operations):
$('*') .resetTransform() .attr('pos', 125) .attr('scale', function(element, index, elements){ return index / elements.length * 5; }) .orderBy('size', true);
align(props)
Align elements to one another
The align() method repackages the native Flash align functionaliity, with a slightly different parameter syntax. Note that align() will soon support aligning to objects, similar to match();
The following example creates and scales some :
$('*') .resetTransform() .randomize({'pos':300, scale:2, rotation:360}) .align('top')
distribute(props, toStage)
Distribute elements relative to one another
The distribute() method repackages the native Flash distribute functionaliity, with a slightly different parameter syntax.
The following example positions, randomizes, then distributes elements horizontally:
$('*') .attr('pos', 30) .randomize({x:400, width:[5, 40]}) .attr('height', function(e){return e.width}) .distribute('horizontal')
space(direction, type)
Space elements relative to one another
The distribute() method repackages the native Flash space functionaliity, with a slightly different parameter syntax.
The following example positions, randomizes, then distributes elements horizontally in a few different ways:
$('*') .attr('pos', 30) .randomize({x:400, width:[5, 40]}) .attr('height', function(e){return e.width}) .space('horizontal') // spaces according to the current collection bounds .space('horizontal', 0) // spaces left-to-right, with a 0px gutter .space('horizontal', true); // spaces to the document bounds (only effective on the root)
match(prop, element)
match elements' dimensions relative to one another
The match() method adds to JSFL's native match functionality by allowing you to choose how element sizes are matched, with a slightly different syntax from the native method.
The following example applies the default match (equivilent to passing true as the 2nd parameter) which is to match to the largest size:
$('*') .randomize('rotation', 360) .match('size', 0)
The following example chooses another element within the collection to match the height to:
$(squares) .randomize({size:50, y:'20'}) .match('height', circle);
toGrid(precision, rounding)
Repositions element positions to round multiples of numbers
The following example aligns all elements to the grid:
$('*').toGrid(0);
The following example creates some squares, randomizes them, then snaps them to the nearest 25 pixels:
makeSquares(3 * 6, 6); $('*') .randomize({pos:25}) .toGrid(25);
The following example uses the Iterators class to align all elements in all library items, across all layers and frames, to the closest pixel:
function alignElements(frame, index, frames, context) { trace('Aliging elements in: ' + context); $('*').toGrid(0); } Iterators.items(true, null, null, alignElements);
Aliging elements in: [object Context dom="library items 02.fla" timeline="Button 2" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="Button 3" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 1" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 1" layer[0]="Layer 1" keyframe[1]=3] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 1" layer[0]="Layer 1" keyframe[2]=8] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 1" layer[1]="Layer 2" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 1" layer[2]="Layer 3" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 1" layer[2]="Layer 3" keyframe[1]=3] Aliging elements in: [object Context dom="library items 02.fla" timeline="Sprite 1" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="Button 1" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="Graphic 1" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="Graphic 2" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="Sprite 2" layer[0]="Layer 1" keyframe[0]=0] Aliging elements in: [object Context dom="library items 02.fla" timeline="MovieClip 3" layer[0]="Layer 1" keyframe[0]=0]
randomize(prop, value)
Randomizes properties of the elements
The randomize() method allows you to randomize the properies of elements by passing numbers, expressions, and ranges as the methods's 2nd argument (see xjsfl.utils.randomizeValue() for more information):
You may also pass in a single Object of property:value pairs, to update multiple properties at once. Note that randomize() does not yet support pseudo properties such as tint, brightness and color.The following example creates some elements, then randomizes various properties using a variety of techniques:
makeSquares(3 * 6, 6); $('*') .attr('x', function(e){ return e.x * 2; }) .randomize({ pos:100, size:[60, 10], rotation:'180', scale:'150%' });
Editing methods
exec(callback, params, scope)
Enters edit mode for each symbol item in the collection, executing a user-supplied function within each one
The following example loops over the current selection, and enters edit mode for each Item, fires an alert box, then eventually returns to the original Document context:
function callback(element, index, param1) { alert(param1 + ' We are now in element ' +index+ ' called "' + element.name + '"'); } var collection = $($selection); collection.exec(callback, 'Hello there!');
This allows you to run custom code within each Library Item without losing your place in the document:
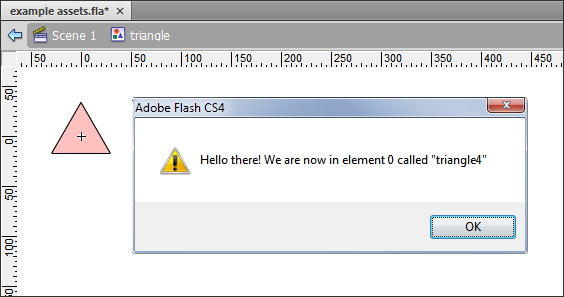
Utility methods
refresh()
Forces the a refreshes of the display after a series of operations
Sometimes after a series of element updates, the stage doesn't update. The refresh() method forces an update.
The following example refreshes the stage after a hypothetical operation that leaves the stage in an invalid state:
$('*') .each(someOperation) .refresh();
Comments are closed.